Question Banks QB Solutions for C++ programs given for class 12 Hsc Computer Science of Maharashtra Board for CS Paper-1.
To download the pdf for Question Banks Solutions of C++ Programs class 12 Computer Science Click below link download button
QB C++ programs solutions class 12 Hsc
Q1. Write a program in C++, to find max of two numbers using if else control structure.
Code:-
#include<iostream>
using namespace std;
int main()
{
int num1, num2, max_no;
cout<<"Enter the Two Numbers: ";
cin>>num1>>num2;
if(num1>num2)
max_no = num1;
else
max_no = num2;
cout<<"\nMaximum of two nos is = "<<max_no;
cout<<endl;
return 0;
}
Q2. Write a program in C++, to find maximum and minimum of two numbers using conditional operator.
Code:-
#include<iostream>
using namespace std;
int main()
{
int num1, num2, max, min;
cout<<"Enter two numbers:";
cin>>num1>>num2;
max = (num1 > num2) ? num1 : num2;
min = (num1 < num2) ? num1 : num2;
cout<<"Maximum between "<<num1<<" and "<<num2<<" is "<<max<<endl;
cout<<"Minimum between "<<num1<<" and "<<num2<<" is "<<min<<endl;
return 0;
}
Q3. Write a program in C++, to find if the given year is leap year or not using if else control structure.
Code:-
#include <iostream>
using namespace std;
int main()
{
int year;
cout << "Enter a year to check: ";
cin >> year;
if (year % 4 == 0)
{
if (year % 100 == 0)
{
if (year % 400 == 0)
cout << year << " is a leap year.";
else
cout << year << " is not a leap year.";
}
else
cout << year << " is a leap year.";
}
else
cout << year << " is not a leap year.";
return 0;
}
To view the execution process you can watch this youtube video and if need then you can subscribe to this channel.
Q4. Write a program in C++, to find factorial of a given number using for loop.
Code:-
#include <iostream>
using namespace std;
int main()
{
int i,fact=1,number;
cout<<"Enter any positive Number: ";
cin>>number;
for(i=1;i<=number;i++)
{
fact=fact*i; //for 5! = 5*4*3*2*1
}
cout<<"Factorial of entered number" <<number<<" is: "<<fact<<endl;
return 0;
}
Note:- Above programs can be executed through the application DevC++, it is available free you can download it from this link. And the write the code using new file option and then press F11 on your computer keyboard to compile and Run the program.
Below is the screen shot of DevC++ code editor window and the console output screen as well for more clearance on it!
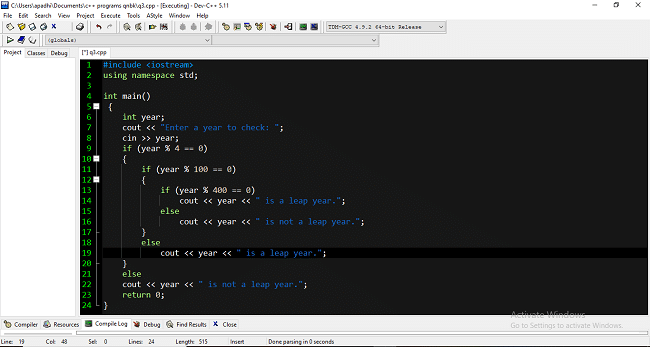
Output console window–
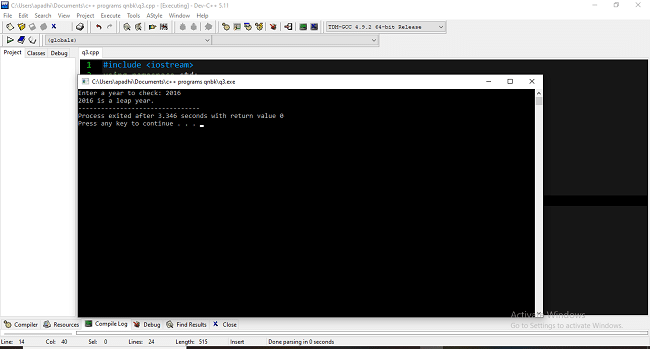
Question Bank of C++ programs solutions class 12 Hsc
Q5. Write a program in C++, to print the sum of first 100 natural numbers using for control structure.
Code:-
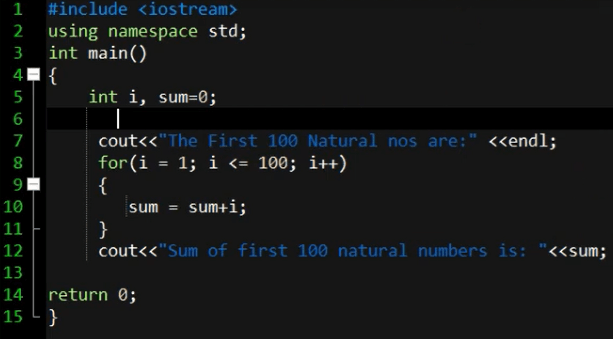
Q6. Write a program in C++, to print first 15 terms of Fibonacci series
Code:-
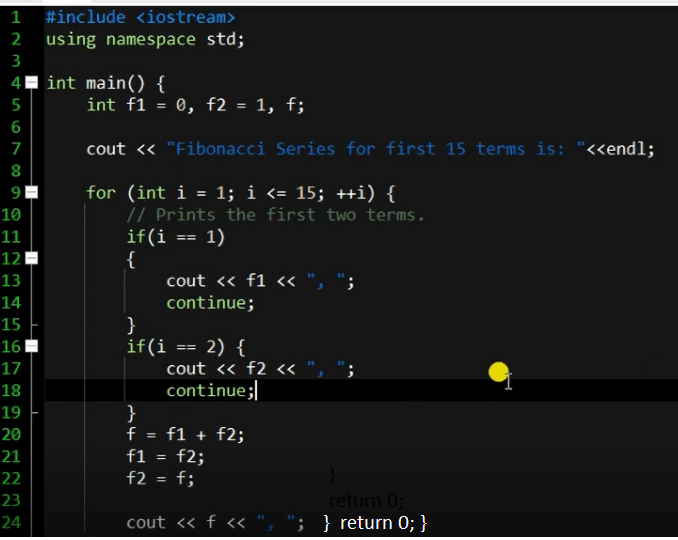
Q7.Write a program in C++, to read array of 10 elements and print its sum.
Code:-
#include<iostream>
using namespace std;
main()
{
int arr[10],i,sum=0;
cout<<"Enter any elements in Array: ";
for(i=0;i<10;i++)
{
cin>>arr[i];
}
cout<<"Sum of all Elements are: ";
for(i=0;i<10;i++)
{
sum=sum+arr[i];
}
cout<<sum;
}
Question Bank Solutions Computer Science Class 12 hsc maharashtra board
Q8.Write a program in C++, to find factorial of a given number using function void fact (int);
Code:-
#include <iostream>
#include <conio.h>
using namespace std;
void fact(int num);
int main(void)
{
int num;
cout<<"Enter the number to find factorial\n";
cin>>num;
fact(num);
getch();
return 0;
}
void fact(int num)
{
int i,f=1;
for(i=1; i<=num; i++)
{
f=f*i;//4!=4*3*2*1
}
cout<<"Factorial of "<<num<<"is:"<<f;
}
Q9. Write an Object Oriented Program in C++, to implement inventory class to calculate total price of number of items purchased.
Code:-
#include <iostream>
using namespace std;
class inventory
{
private:
int no_of_items;
float total_price;
float price;
public:
void getData(void);
void calculate(void);
void putData(void);
};
void inventory::getData(void)
{
cout << "Enter number of items to be purchased: "<<endl ;
cin >> no_of_items;
cout << "Enter Price of each items purchased ";
cin >> price;
}
void inventory::calculate(void)
{
total_price=(float)no_of_items*price;
cout<<"Total price of number of Items Purchased are:"<<total_price;
}
void inventory::putData(void){
cout << "Items Purchasing details:"<<endl;
cout << "Number of Items purchased is:"<< no_of_items <<endl;
cout<<"Price Per items is:" <<price<<endl;
calculate();
}
int main()
{
inventory in;
in.getData();
in.putData();
return 0;
}
Q10. Write an Object Oriented Program in C++, to find the GCD of two given numbers.
Code:-
#include <iostream>
using namespace std;
int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
int main() {
int a = 225, b = 45;
cout<<"GCD of TWO NUMBERS"<< a <<" and "<< b <<" is "<< gcd(a, b);
return 0;
}
Q11. Implement a class fact, to find the factorial of a given number.
Code:-
#include<iostream>
using namespace std;
class fact
{
private:
int i,f=1,number;
public:
void input()
{
cout<<"Enter any positive Number: ";
cin>>number;
}
void calculate()
{
for(i=1;i<=number;i++)
{
f=f*i; //for 5! = 5*4*3*2*1
}
}
void display()
{
cout<<"Factorial of entered number" <<number<<" is: "<<f<<endl;
}
};
int main() {
fact f;
f.input();
f.calculate();
f.display();
return 0;
}
Q12. Write an Object Oriented Program in C++, to implement circle class to find area and circumference of a circle using functions void area(), void circum().
Code:-
#include<iostream>
using namespace std;
class Circle
{
public:
float radius, area_of_circle,circumference;
void getRadius()
{
cout << "Enter radius of a circle:";
cin >> radius;
}
void area()
{
area_of_circle = 3.14 * radius * radius;
}
void circum()
{
circumference= 2 * 3.14 * radius;
}
void display()
{
cout << "Area of circle is:" << area_of_circle<<endl;
cout << "Circumference of circle is:" << circumference;
}
};
int main() {
Circle cir;
cir.getRadius();
cir.area();
cir.circum();
cir.display();
return 0;
}
Q13. Write a program in C++ to find the no of occurrences of character ‘a’ in the given string.
Code:-
#include<iostream>
using namespace std;
int main()
{
int i,count=0;
char ch[50]="claass12computerabc";
for(i=0;ch[i]!='\0';i++)
{
if(ch[i]=='a')
count++;
}
if(count==0)
{
cout<<"\nEntered character a in above string not found."<<endl;
}
else
{
cout<<"Number of Occurrences for particular character a is : "<<count<<" times."<<endl;
}
return 0;
}
Q14. Write a Program in C++, to reverse a given string.
Code:
#include<iostream>
#include<string.h>
using namespace std;
int main()
{
char str[100], temp;
int i=0, j;
cout<<"\n Enter String to be reversed: ";
gets(str);
i=0;
j=strlen(str)-1;
while(i<j)
{
temp=str[i];
str[i]=str[j];
str[j]=temp;
i++;
j--;
}
cout<<"\n Reversed String is : "<<str;
return 0;
}
Q15. Implement class temperature to convert degree Fahrenheit (F) to degree Celsius (C).
Using formulae C =( F -32/9)*5.
Code:-
#include<iostream>
using namespace std;
class Temperature
{
float f,c;
public:
float getdata();
void display(void);
};
float Temperature :: getdata()
{
cout<<"Enter temperature in degree farenheit to convert it for celsius:- ";
cin>>f;
c = ((f-32)/9)*5; //formulae given t convert
return(c);
}
void Temperature :: display(void)
{
float cel;
cel=getdata();
cout<<"Converted to Degree Celsius = "<<cel;
}
int main()
{
Temperature c;
c.display();
return 0;
}
Q16. Write a program in C++, to find the largest number in an array of 10 integers.
Code:-
#include<iostream>
using namespace std;
int main()
{
int arr[10], max=10, larg, i;
cout<<"Enter 10 Array Elements: ";
for(i=0; i<max; i++)
cin>>arr[i];
for(i=1; i<=max; i++)
{
if(larg<arr[i])
larg = arr[i];
}
cout<<"\nLargest Number = "<<larg;
cout<<endl;
return 0;
}
Q17. Write a program in C++, to check if the given number is a prime number .
Code:-
#include <iostream>
using namespace std;
int main()
{
int n, i, cnt = 0;
cout << "Enter any number n: ";
cin>>n;
for (i = 1; i <= n; i++)
{
if (n % i == 0)
{
cnt++;
}
}
if (cnt == 2)
{
cout << n <<" is a Prime number" << endl;
}
else
{
cout <<n<< " is not a Prime number" << endl;
}
return 0;
}
Q18. Write a program in C++, to calculate xy , using the function void power (int, int);
#include<iostream>
using namespace std;
void power(int x, int y);
int main(void)
{
double x;
int y;
cout<<"Enter the number to find the power of:"<<endl;
cin>>x;
cout<<"Enter raise to the power of above no"<<endl;
cin>>y;
power(x, y);
return 0;
}
void power(int x, int y)
{
int i,z;
for( i=0;i<y;i++)
{
z=z*x;
}
for(i=0;i>y;i--)
{
z=z/x;
}
cout<<z;
}
Q19. Write a program in C++, to count the number of words in a line of text?
Code:
#include<iostream>
using namespace std;
int main()
{
char str[500];
int i=0, ch=0, cnt=0;
cout<<"Enter the String: ";
gets(str);
while(str[i]!='\0')
{
if(str[i]==' ')
{
if(ch!=0)
cnt++;
ch=0;
}
else
{
ch++;
}
i++;
}
if(ch!=0)
cnt++;
cout<<"\nTotal Number of Words in above line is = "<<cnt;
cout<<endl;
return 0;
}
Q20. Write a program in C++, to swap two integers using function void swap (int &, int&);
Code:
#include<iostream>
using namespace std;
void swap(int &,int &);
int main()
{
int a,b;
cout<<"Enter the two Numbers to swap"<<endl;
cin>>a>>b;
cout<<"you entered a="<<a<<" b="<<b;
cout<<"\nAfter swapping of two numbers: we get"<<endl;
swap(a,b);
return 0;
}
void swap(int &x,int &y)
{
int temp;
temp=x;
x=y;
y=temp;
cout<<"a="<<x<<" b="<<y;
}
Question Bank Solutions for HTML Program code for class 12 HSC Computer science CS paper – 1 Read More
Bohat mast kaam karte ho aap bhai