This article is all about the solutions of Skill oriented practicals given in your class 12 IT textbook of chapter Advanced Javascript as “HSC IT Advanced JavaScript SOP Practicals Textbook Solutions”.
SOP 1. Skill Oriented Practicals – 01 of textbook HSC IT Maharashtra Board class 12 Science 2024
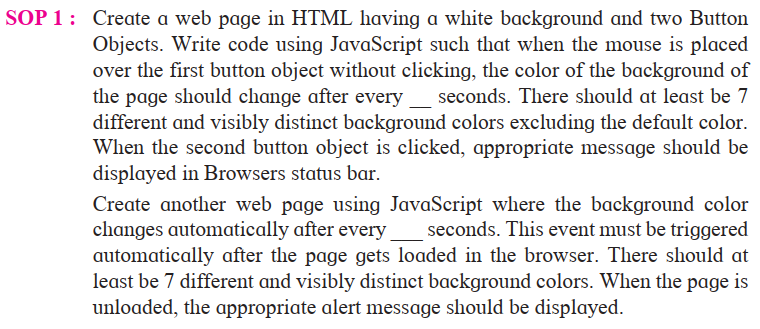
Solution Code:
<!DOCTYPE html>
<html>
<head>
<title>7 Different Colors</title>
</head>
<body>
<script type="text/javascript">
var colors = new Array("blue", "yellow", "red", "green", "orange","cyan","indigo");
var i=0;
function changeColor()
{
document.body.style.backgroundColor = colors[i];
i++;
if(i>colors.length)
{
i=0;
}
window.setTimeout("changeColor()",2000);
}
function disp_mesg_status()
{
window.status="All Seven Colors Displayed";
}
</script>
<form>
<input type="button" name=btn_color value="changecolors" onmouseover="changeColor()">
<input type="button" name="btn_mesg" value="Display Message" onclick="disp_mesg_status()">
</form>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body onload="changeColor()">
<script type="text/javascript">
var colors = new Array("blue", "yellow", "red", "green", "orange","cyan","indigo");
var i=0;
function changeColor()
{
document.body.style.backgroundColor = colors[i];
i++;
if(i>colors.length)
{
i=0;
}
window.setTimeout("changeColor()",1500);
}
window.onbeforeunload = function()
{
var msg = "Seven Different Colors Displayed";
return msg;
}
</script>
</body>
</html>
Note: For the above code unload event is not taken as it not working on browsers of latest versions like operamini, IE, Firefox, chrome etc..so instead onbeforeunload event has been taken.
SOP 2 HSC IT Advanced JavaScript SOP Practicals Textbook Solutions
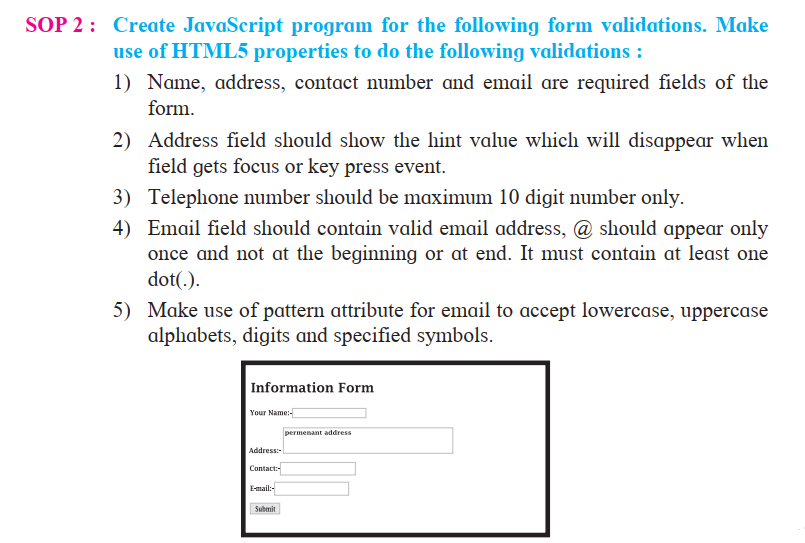
Solution Code:
<!DOCTYPE html>
<html>
<head>
<title>Sop 2 JAVaScript</title>
</head>
<body>
<h1>Information Form</h1>
<form name="f1">
Your Name
<input type="text" name="txt_name">
<br>
<br>
Address
<textarea name="txt_address" placeholder="Permanent Address"/></textarea>
<br>
<br>
Contact
<input type="tel" name="telephone" maxlength="10">
<br><br>
Email
<input type="email" name="txt_email" pattern="[A-Z a-z]{0-9}-[@]{1}-[.]{1}">
<br>
<br>
<input type="button" name="b1" value="submit" onclick="validate_email()">
</form>
</body>
<script type="text/javascript">
function validate_email()
{
var x=f1.txt_email.value;
var at_pos=x.indexOf("@");
var last_pos=x.lastIndexOf("@");
var firstdot_pos=x.indexOf(".");
var dot_pos=x.lastIndexOf(".");
if (at_pos<1||dot_pos<at_pos+2||dot_pos+2>=x.length||firstdot_pos<at_pos||at_pos<last_pos)
{
alert("Not an Valid email address");
f1.txt_email.focus();
}
else
{
alert("Valid Email Address");
return true;
}
}
</script>
</html>
Sop3: IT Practical Solutions of Javascript chapter3
Create event driven javascript program for the following. Make use of appropriate variables., Javascript inbuilt variables string functions and control structures.
To accept string form user and count number of vowels in the given string.
Code Solution:
<!DOCTYPE html>
<html>
<head>
<title>Sop 3 JAVasript Count vowels</title>
</head>
<body>
<form name="form1">
<h1>Enter the String whose vowel isto be counted</h1>
<input type="text" name="text1">
<input type="button" name="btn_checkvowel" value="Click to count" onclick="count()">
</form>
<script type="text/javascript">
function count()
{
var i,ch,str,counter=0;
str=form1.text1.value;
for(i=0;i<str.length;i++)
{
ch=str.charAt(i);
if(ch=='A'||ch=='a'||ch=='e'||ch=='E'||ch=='i'||ch=='I'||ch=='o'||ch=='O'||ch=='u'||ch=='U')
counter++;
}
alert("Number of Vowels in Entered String is:"+counter);
}
</script>
</body>
</html>
SOP 4. HSC IT SOP Chapter 3 Advanced Javascript
Create event driven javascript program for the following. Make use of appropriate variables., Javascript inbuilt variables string functions and control structures.
To accept string from user and reverse the given string and check whether it is palindrome or not.
Solution Code:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript SOP 4 Example</title>
</head>
<body>
<form name="f1">
Enter the string to check it is palindrome or not!
<br>
<input type="text" name="t1">
<br>
<br>
<input type="button" name="check_palin" value="Check String" onclick="chk_palindrome()">
</form>
<script type="text/javascript">
function chk_palindrome()
{
var str,str_case,i,len;
str=f1.t1.value;
str_case=str.toLowerCase();
len=str_case.length;
var p=1;
for(i=0;i<len/2;i++)
{
if(str_case.charAt(i)!=str_case.charAt(len-1-i))
{
p=0;
break;
}
}
if(p==1)
{
alert("Entered string is Palindrome");
}
else
{
alert("Entered string is Not a Palindrome")
}
}
</script>
</body>
</html>
SOP 5. Javascript class 12 HSC IT 2022
Create a event driven javascript program to convert temperature to and from Celsius, Fahrenheit.
Code Solution:
<!DOCTYPE html>
<html>
<head>
<title>SOP 5 Javascript</title>
<body>
<h2>JavaScript code to convert Celcius to Fahrenhiet or vice-versa</h2>
<br>
<p>Enter the Temperature</p>
<p><input type="text" id="txt_celsius" onkeyup="convert('C')"> Temperature in degree Celsius</p>
<br>
<p><input type="text" id="txt_fah" onkeyup="convert('F')"> Temperature in degree Fahrenheit</p>
<script>
function convert(temperature) {
var t;
if (temperature == "C") //Celsius to fahrenit
{
t = document.getElementById("txt_celsius").value * 9 / 5 + 32;
document.getElementById("txt_fah").value = Math.round(t);
}
else //fahrenirt to celsius
{
t = (document.getElementById("txt_fah").value -32) * 5 / 9;
document.getElementById("txt_celsius").value = Math.round(t);
}
}
</script>
//Note:-you can remove math.round function if you need answer in decimal
</body>
</html>
SOP 6 Advanced Javascript solution of class 12 IT
Create javascript program which compute average marks of students accepts from user and display the corresponding grades.
Solution Code:
<!DOCTYPE html>
<html>
<head>
<title>Sop 6 Javascript program</title>
</head>
<body>
<h1>
Javascript program to compute average marks of students
</h1>
<form name="form1">
<p>Enter Marks of subjects of students
<br><br>
English Marks
<input type="text" name="txt_eng">
<br><br>
Physics Marks
<input type="text" name="txt_phy">
<br><br>
Chemistry Marks
<input type="text" name="txt_chem">
<br><br>
IT Marks
<input type="text" name="txt_it">
<br><br>
Maths Marks
<input type="text" name="txt_maths">
<br><br>
Biology Marks
<input type="text" name="txt_bio">
<br><br>
<input type="submit" value="Calculate Average & print grade" onclick="calculate_grade()">
</form>
<script type="text/javascript">
function calculate_grade()
{
var m1,m2,m3,m4,m5,m6,avg;
m1=parseInt(form1.txt_eng.value);
m2=parseInt(form1.txt_phy.value);
m3=parseInt(form1.txt_chem.value);
m4=parseInt(form1.txt_it.value);
m5=parseInt(form1.txt_maths.value);
m6=parseInt(form1.txt_bio.value);
avg=(m1+m2+m3+m4+m5+m6)/6; //computes the average of six subjects of students
alert("Average marks of students for six subjects is:"+ avg);
if(avg>=91 && avg<=100)
{
grade = 'A';
}
else if(avg>=81 && avg<=90)
{
grade = 'B';
}
else if(avg>=71 && avg<=80)
{
grade = 'C';
}
else if(avg>=61 && avg<=70)
{
grade = 'D';
}
else if (avg>=35 && avg<=60)
{
grade = 'F';
}
alert ("Grade of the student is: "+ grade);
}
</script>
</body>
</html>
SOP7 Javascript solution HSC IT
Write a javascript function to get difference between two dates in days. Create a page in HTML that contains input box to accept date from user. The input boxes should be used by users to enter their date-of-birth in the format dd-mm-yyyy . Do not make use of drop down boxes.
Advanced Web Designing SOP Solutions Read More
Solutions code:
Here is the Full playlist for Skill Oriented Practicals code demonstration performing with explanation and output of chapter 3 advanced javascript.
Subscribe for more videos on class 12th HSC IT chapters of science commerce Arts
HSC IT TextBook solutions with chapter Explanation 2022 Read More.
Advanced Web Designing HSC IT Notes Read More
Emerging Technologies chapter exercise solution Read More
HSC IT Class 12 Chapter 2 (Introduction to SEO) Exercise Solutions Read More
IT Sample Model Paper 2024 HSC Maharashtra Board Read More
HSC IT SOP(Skill Oriented Practical Solutions) Class 12 Read More
Advanced Web Designing SOP Solutions Read More
Chapter Wise Weightage marks distribution Class 12 HSC Science Maharashtra Board all Subjects click to read
sir we are not able to copy the program from here
ohk…but you can refer the code from site…and type it on the editor…which will able to put hands on good code practice…..its my opinion to you as a teacher…..
But then if you still need the code for copy and paste..let me know….
actually my output is not coming. thats why i wanted the code.
please let me know which javascipt sop code you need because i have listed almost all..1st one is not listed..
Sir please upload 11th IT
ohk..will upload on youtube and here as well..which is more preferrable..
Pingback: hsc it sop practical solutions science commerce: HSC IT SOP Practical Program Solutions | Techniyojan
Pingback: Can I run HTML codes on a mobile phone via any software? | Techniyojan
Pingback: 12th IT ServerSide Scripting PHP Practical Solutions Hsc Maharashtra board new syllabus 2021 | Techniyojan
Pingback: Class 12th HSC IT Notes all chapters textbook Exercise solutions MH Board
i tried running this code but its giving me error as invalid character do we need to install any javascript libraries or it will be ok with html-notepad
no need and its ok with notepad
Sop6 (12th IT javascript) not working…
whats the issue eror
Pingback: 12th Sci IT SOP Solutions All Chapters HSC Mah Board | Techniyojan
Solution of SOP7 is not here..!
please upload it..!
Pingback: Advanced JavaScript Exercise Solutions HSC IT | Techniyojan
Pingback: Advanced Web Designing class 12 (IT) notes Information Technology chapter1 | HSC Maharashtra board 2020